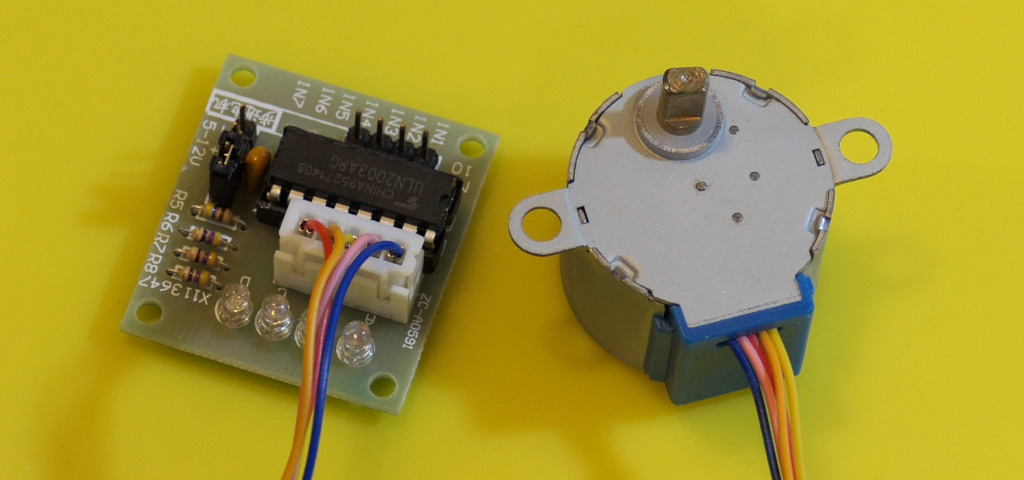
Stepper motors can move back and forth and lock into fixed positions, or steps, around the axis.
Stepper motors are common in machines that require precise and stable movement, like 3D printers and CNC machines.
Usually you will connect a stepper motor to your microcontroller by means of a stepper motor driver board, which has all the circuitry for controlling the motor. Then for you to control the motor, you just need to provide power and a signal to trigger the motor's intended position.
Small unipolar stepper motors like the one pictured often use a driver based on the ULN2003A or similar chip. This particular board uses a total of six pins: four pins to control the stepper, plus a 5V-12V power source sufficient for driving the motor, and GND.
The four input pins are connected to four GPIO pins on a microcontroller. The microcontroller controls the motor's step sequence by turning combinations of these pins on and off. The combinations and sequences vary between different motors, so writing the code to control a stepper motor at this level can be a tedious task.
Arduino code
Arduino includes a library called Stepper.h that supports many common hobbyist stepper motor drivers. Others in the community have also written libraries for specific drivers. These libraries hide the details of communicating with the stepper motor driver, so you can control it with simple commands like setSpeed
or step
.
#include <Stepper.h>
// Total number of steps per rotation.
// This may be different for your motor.
const int totalSteps = 2038;
// Define how the pins on the driver board
// are connected to the pins on the Uno board.
const int IN1 = 8;
const int IN2 = 9;
const int IN3 = 10;
const int IN4 = 11;
// Define a Stepper variable that we'll call "motor".
// Note: The step sequence of pins may differ
// for your particular motor.
Stepper motor = Stepper(totalSteps, IN1, IN3, IN2, IN4);
void setup() {
}
void loop() {
// Turn clockwise
motor.setSpeed(100);
motor.step(totalSteps);
delay(1000);
// Turn counterclockwise
motor.setSpeed(700);
motor.step(-totalSteps);
delay(1000);
}
Tags
- Log in to post comments